C is a general-purpose programming language that was developed in the early 1970s by Dennis Ritchie at Bell Labs.
It is a high-level language that provides low-level access to system-level resources, making it popular for writing operating systems, compilers, and other system software.
C is a procedural language, which means that it follows a top-down approach to programming.
It uses a set of functions and procedures to perform tasks, and these functions can be reused in different parts of the program.
C is also a compiled language, which means that the source code is first compiled into machine code before it can be executed.
C has a rich set of data types and operators that allow programmers to work with variables and data structures in a flexible manner.
It also has control structures such as loops and conditional statements, which enable programmers to write complex algorithms.
C has had a significant impact on the development of modern computing and has influenced many other programming languages.
It is widely used in the development of operating systems, embedded systems, and software that requires high performance and low-level access to system resources.
History of c
C was developed by Dennis Ritchie at Bell Labs in the early 1970s as an evolution of the B programming language. Ritchie wanted to create a language that could be used to write system software for the Unix operating system, which was also being developed at Bell Labs at the time.
C quickly gained popularity among programmers and became the de facto language for system programming.
In the 1980s, the American National Standards Institute (ANSI) standardized the C language, which led to the development of the ANSI C standard.
The C language continued to evolve, with new features and capabilities being added over time.
C's popularity continued to grow throughout the 1990s and 2000s, and it remains a popular language today.
Its influence can be seen in many modern programming languages, including C++, Java, Python, and many others.
C is still widely used for system programming, as well as for embedded systems, device drivers, and other applications that require direct access to system resources.
Features of C
C is a versatile language that has many features, including:
1. Low-level access: C allows programmers to have low-level access to system resources, making it useful for system programming and developing operating systems, device drivers, and other low-level software.
2. Portability: C code can be compiled and run on different platforms, making it a portable language.
This is because C compilers are available for a wide range of hardware and operating systems.
3. Efficiency: C is a compiled language, which means that it can be optimized to run efficiently on a given hardware platform.
This makes it useful for developing applications that require high performance.
4. Modularity: C supports modular programming, which allows programmers to break down a large program into smaller, more manageable modules.
These modules can be developed and tested separately and then combined into a larger program.
5. Rich data types: C supports a wide range of data types, including integers, characters, floating-point numbers, arrays, structures, and pointers.
This makes it useful for developing applications that require complex data structures.
6. Powerful operators: C supports a wide range of operators, including arithmetic, bitwise, logical, and relational operators.
This makes it useful for developing algorithms and performing complex computations.
7. Control structures: C supports a range of control structures, including if-else statements, for loops, while loops, and switch statements.
These control structures enable programmers to write complex algorithms and control the flow of program execution.
8. Standard library: C comes with a standard library that provides a wide range of functions for performing common tasks, such as input/output operations, string manipulation, and memory allocation.
C Characters Set
The C character set is the set of characters that are recognized by the C programming language.
It includes the following:
1. Uppercase and lowercase letters: A to Z and a to z, respectively.
2. Digits: 0 to 9.
3. Punctuation marks: . (dot), , (comma), ; (semicolon), : (colon), ! (exclamation mark), ? (question mark), ' (single quote), " (double quote), and - (dash).
4. Mathematical operators: + (plus), - (minus), * (multiply), / (divide), and % (modulus).
5. Brackets and parentheses: (, ), {, and }.
6. Special characters: \ (backslash), which is used to escape special characters and create escape sequences, and the space character.
The C character set is based on the ASCII character set, which is a standard character encoding system that assigns unique numbers to each character. The ASCII character set includes 128 characters, which are represented by the numbers 0 to 127. In C, each character is represented by its corresponding ASCII code. For example, the character 'A' has the ASCII code 65, 'B' has the ASCII code 66, and so on.
Keywords and Identifiers in C
Keywords and identifiers are two important concepts in the C programming language.
Keywords:
Keywords are reserved words that have special meaning in the C language.
They cannot be used as variable names or identifiers.
Examples of keywords in C include if, else, for, while, switch, and return.
There are 32 keywords in the standard C language.
Identifiers:
An identifier is a name that is used to identify a variable, function, or other user-defined item in a C program.
Identifiers can be composed of letters, digits, and underscores, and must begin with a letter or underscore.
Identifiers are case sensitive, which means that uppercase and lowercase letters are treated as different characters.
Examples of valid identifiers in C include num, sum_total, and calculate_area.
Identifiers should be chosen carefully to ensure that they are descriptive and meaningful.
It is also important to avoid using keywords as identifiers, as this can lead to errors and make the code difficult to read.
Keywords are reserved words that have a specific meaning in the C language.
They cannot be used as identifiers or variable names. Some common C keywords include:
`auto`, `break`, `case`, `char`, `const`, `continue`, `default`, `do`, `double`, `else`, `enum`, `extern`,
`float`, `for`, `goto`, `if`, `int`, `long`, `register`, `return`, `short`, `signed`, `sizeof`, `static`, `struct`,
`switch`, `typedef`, `union`, `unsigned`, `void`, `volatile`, `while`.
Identifiers, on the other hand, are names given to variables, functions, or user-defined data types.
They are created by the programmer and should be descriptive and meaningful.
Identifiers must follow certain rules in C, such as:
- They must start with a letter (either uppercase or lowercase) or an underscore (_).
- They can be followed by any combination of letters, digits, or underscores.
- They cannot be the same as a C keyword.
- They are case sensitive.
Examples of valid C identifiers include:
`count`, `sum`, `x`, `my_var`, `MyStruct`, `functionName`.
It is important to choose meaningful and descriptive identifiers in order to write readable and maintainable code.
Data Types in C
Data types in C are used to define the type of data that a variable can hold.
They specify the size and layout of the variable's memory, the range of values that can be stored, and the set of operations that can be performed on the variable.
C has several built-in data types, including:
1. Integers: Integers are whole numbers without a fractional component. They can be signed or unsigned, meaning they can be positive or negative. C supports four integer data types: char, short, int, and long, which have different ranges and sizes.
2. Floating-point numbers: Floating-point numbers are numbers with a fractional component. They can be represented in scientific notation and have a certain amount of precision. C supports two floating-point data types: float and double.
3. Characters: Characters are single letters or symbols. They are represented by the char data type, which is used to store individual characters or small strings of characters.
4. Booleans: Booleans are data types that can hold one of two values: true or false. In C, the bool data type is not built-in, but can be defined using typedef.
5. Enumerated types: Enumerated types are user-defined data types that consist of a set of named constants. They are often used to define a set of related values that are used throughout a program.
6. Void: Void is a special data type that represents the absence of a value. It is often used to indicate that a function does not return a value or to define generic pointers.
Each data type in C has its own set of properties and limitations. Choosing the right data type for a variable is important to ensure that the program is efficient and correct.
OR
C supports several data types that can be used to declare variables and functions. These data types include:
1. Integers: Used to represent whole numbers. C supports several integer data types, including char, short, int, long, and long long. These data types differ in the range of values they can represent and the amount of memory they occupy.
2. Floating-point numbers: Used to represent decimal numbers. C supports two floating-point data types: float and double. Float variables occupy 4 bytes of memory, while double variables occupy 8 bytes.
3. Characters: Used to represent individual characters. The char data type is used to declare character variables.
4. Booleans: Used to represent true/false values. C does not have a built-in boolean data type, but boolean values can be represented using integers or macros.
5. Pointers: Used to store memory addresses. A pointer variable stores the memory address of another variable or function. Pointers are often used to pass variables and arrays to functions by reference.
6. Arrays: Used to store multiple values of the same data type. An array is a collection of variables of the same data type, which are stored in contiguous memory locations.
7. Structures: Used to store multiple values of different data types. A structure is a user-defined data type that can contain variables of different data types.
8. Unions: Used to store different types of data in the same memory location. A union is a user-defined data type that can store different variables of different data types in the same memory location.
C also allows programmers to create their own user-defined data types using structures, unions, and typedefs.
Constant Vriables and their decleration in C
In C, a constant variable is a variable whose value cannot be changed once it has been assigned. Constant variables are useful for defining values that should not be modified during the execution of a program, such as mathematical constants or configuration values.
To declare a constant variable in C, the const keyword is used. The general syntax for declaring a constant variable is as follows:
```c
const data_type variable_name = value;
```
Here, `data_type` is the data type of the variable, `variable_name` is the name of the variable, and `value` is the initial value of the variable. The `const` keyword tells the compiler that the variable is a constant and cannot be modified.
For example, to declare a constant integer variable named `PI` with the value 3.14159, the following code can be used:
```c
const int PI = 3.14159;
```
Once a constant variable has been declared, attempts to modify its value will result in a compiler error. For example, the following code will generate a compiler error:
```c
PI = 3.14; // Compiler error: assignment of read-only variable
```
Constant variables can also be used in expressions, just like regular variables. For example, the following code is valid:
int radius = 5;
float circumference = 2 * PI * radius;
In this example, the constant variable `PI` is used in an expression to calculate the circumference of a circle with a radius of 5.
Formatted input output in c
Formatted input/output (I/O) in C is a way of reading input and displaying output using a specific format. Formatted I/O is useful for presenting data in a specific way or for reading input that is formatted in a specific way. The formatted I/O functions in C are part of the standard I/O library, which is included in the header file `stdio.h`.
The two main functions for formatted output in C are `printf()` and `sprintf()`. The `printf()` function is used to display output to the console, while the `sprintf()` function is used to format output as a string.
The general syntax for the `printf()` function is as follows:
```c
printf("format string", argument1, argument2, ...);
```
Here, `"format string"` is a string that specifies how the output should be formatted, and `argument1`, `argument2`, etc. are the values to be printed. The format string can contain format specifiers, which are special characters that are replaced with the values of the corresponding arguments. For example, the format specifier `%d` is used to display an integer value.
Here is an example of using the `printf()` function to display output in a specific format:
```c
int age = 25;
float height = 1.75;
printf("I am %d years old and %.2f meters tall\n", age, height);
```
In this example, the format string `"I am %d years old and %.2f meters tall\n"` contains two format specifiers: `%d` for the integer variable `age` and `%.2f` for the floating-point variable `height`. The `%.2f` format specifier specifies that the floating-point value should be displayed with two decimal places.
The general syntax for the `sprintf()` function is similar to `printf()`, but the output is formatted as a string instead of being printed to the console:
```c
char buffer[50];
int value = 42;
sprintf(buffer, "The value is %d", value);
```
In this example, the `sprintf()` function formats the string `"The value is %d"` with the integer variable `value`, and stores the resulting string in the character array `buffer`.
Formatted input in C is done using the `scanf()` function. The `scanf()` function reads input from the console and stores it in variables, using a format string to specify the format of the input. The general syntax for the `scanf()` function is as follows:
```c
scanf("format string", &variable1, &variable2, ...);
```
Here, `"format string"` is a string that specifies the format of the input, and `&variable1`, `&variable2`, etc. are pointers to the variables where the input should be stored. The format string can contain format specifiers, just like in `printf()`, to specify the type of input to be read.
Here is an example of using the `scanf()` function to read input in a specific format:
```c
int age;
float height;
printf("Enter your age and height: ");
scanf("%d %f", &age, &height);
printf("You are %d years old and %.2f meters tall\n", age, height);
```
In this example, the format string `"%d %f"` specifies that two values should be read: an integer for the variable `age` and a floating-point number for the variable `height`. The values entered by the user are read from the console and stored in the variables `age` and `height`, and then displayed using the `printf()` function.
C operators
Operators in C are symbols or keywords that represent a specific operation. C supports a wide range of operators, which can be classified into several categories based on their functionality. Here is a brief overview of the operators in C:
1. Arithmetic operators: These operators are used to perform mathematical operations, such as addition, subtraction, multiplication, and division. The arithmetic operators in C include `+` (addition), `-` (subtraction), `*` (multiplication), `/` (division), and `%` (modulus).
2. Assignment operators: These operators are used to assign a value to a variable. The assignment operator in C is `=`.
3. Relational operators: These operators are used to compare two values and determine their relationship. The relational operators in C include `==` (equal to), `!=` (not equal to), `<` (less than), `>` (greater than), `<=` (less than or equal to), and `>=` (greater than or equal to).
4. Logical operators: These operators are used to perform logical operations, such as AND, OR, and NOT. The logical operators in C include `&&` (logical AND), `||` (logical OR), and `!` (logical NOT).
5. Bitwise operators: These operators are used to perform operations on individual bits of binary numbers. The bitwise operators in C include `&` (bitwise AND), `|` (bitwise OR), `^` (bitwise XOR), `~` (bitwise NOT), `<<` (left shift), and `>>` (right shift).
6. Increment and decrement operators: These operators are used to increase or decrease the value of a variable by 1. The increment operator in C is `++`, and the decrement operator is `--`.
7. Conditional operator: This operator is used to perform a conditional operation based on a boolean expression. The conditional operator in C is `?:`.
8. Sizeof operator: This operator is used to determine the size of a data type in bytes. The sizeof operator in C is `sizeof()`.
9. Comma operator: This operator is used to separate two or more expressions in a single statement. The comma operator in C is `,`.
These are the main categories of operators in C. By understanding these operators, you can perform a wide range of operations in your C programs.
Arrays
In C, an array is a collection of similar data items stored in contiguous memory locations. It is a data structure that allows us to store multiple values of the same data type in a single variable. The values in an array can be of any data type, such as int, float, char, etc.
An array in C is declared by specifying the data type of the elements it will contain, followed by the array name and the size of the array in square brackets []. For example, to declare an array of integers with 5 elements, we use the following syntax:
```
int myArray[5];
```
This will create an array called "myArray" that can hold 5 integers.
We can initialize the array at the time of declaration by providing a comma-separated list of values enclosed in curly braces. For example, to initialize the array "myArray" with the values 1, 2, 3, 4, and 5, we use the following syntax:
```
int myArray[5] = {1, 2, 3, 4, 5};
```
To access the elements of an array, we use the array name followed by the index of the element enclosed in square brackets. The index of the first element in an array is always 0. For example, to access the third element of the array "myArray", we use the following syntax:
```
int x = myArray[2];
```
This will assign the value of the third element (which is 3) to the variable "x".
Arrays are very useful in C, as they allow us to store and manipulate large amounts of data efficiently.
In C, there are two main types of arrays: one-dimensional arrays and multidimensional arrays.
1. One-dimensional array:
A one-dimensional array is a collection of data elements of the same data type, arranged in a linear sequence. It can be thought of as a single row or column of elements. To declare a one-dimensional array, we use the following syntax:
```
data_type array_name[array_size];
```
For example, to declare an array of integers with 5 elements, we use the following syntax:
```
int myArray[5];
```
This will create an array called "myArray" that can hold 5 integers.
2. Multidimensional array:
A multidimensional array is an array of arrays. It is used to store data in a tabular form, where data is arranged in rows and columns. A two-dimensional array can be thought of as a matrix, where each element is identified by its row and column position. To declare a multidimensional array, we use the following syntax:
```
data_type array_name[array_size1][array_size2];
```
For example, to declare a two-dimensional array of integers with 3 rows and 4 columns, we use the following syntax:
```
int myArray[3][4];
```
This will create an array called "myArray" that can hold 3 rows and 4 columns of integers.
To access the elements of a multidimensional array, we use the row and column indices enclosed in square brackets. For example, to access the element in the second row and third column of the array "myArray", we use the following syntax:
```
int x = myArray[1][2];
```
This will assign the value of the element at row 1 and column 2 (which is the third element of the second row) to the variable "x".
In summary, one-dimensional arrays are used to store a linear sequence of data elements, while multidimensional arrays are used to store data in a tabular form, where data is arranged in rows and columns.
Initialization of Array and Accessing the elements of array
Initialization of an array in C can be done at the time of declaration or later during the execution of the program. Here are some examples:
1. Initialization at the time of declaration:
```
int arr[5] = {1, 2, 3, 4, 5};
```
This initializes an integer array of size 5 with the values 1, 2, 3, 4, 5.
2. Partial initialization:
```
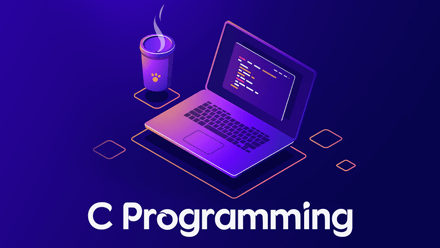
int arr[5] = {1, 2};
```
This initializes the first two elements of the array with values 1 and 2, and the rest are automatically initialized to 0.
3. Accessing array elements:
We can access individual elements of an array by using the index of the element. The first element of an array is at index 0, the second at index 1, and so on. Here is an example:
```c
int arr[5] = {1, 2, 3, 4, 5};
printf("Element at index 2 is %d", arr[2]);
```
This will print "Element at index 2 is 3" because the element at index 2 of the array is 3.
4. Changing the value of array elements:
We can also change the value of individual elements of an array by using the index of the element. Here is an example:
```c
int arr[5] = {1, 2, 3, 4, 5};
arr[2] = 10;
printf("New value at index 2 is %d", arr[2]);
```
This will print "New value at index 2 is 10" because we changed the value of the element at index 2 from 3 to 10.
In summary, we can initialize an array at the time of declaration or later during the execution of the program. We can access individual elements of an array using the index of the element, and we can change the value of an element by assigning a new value to it using its index.
Character array (String)
In C, a string is simply an array of characters terminated by a null character (`\0`). This means that a string is stored as a character array, but the last character in the array is always a null character to indicate the end of the string.
Here is an example of a string in C:
```c
char str[6] = "hello";
```
In this example, we create a character array called `str` with a length of 6 (including the null character), and initialize it with the string "hello". Note that we include the null character in the array explicitly.
We can also initialize a string using a string literal:
```c
char str[] = "world";
```
In this case, the compiler automatically creates a character array of length 6 (including the null character) and initializes it with the string "world". The array size is determined based on the length of the string literal.
To access individual characters in a string, we can use array indexing:
```c
char str[] = "hello";
printf("%c\n", str[0]); // prints 'h'
printf("%c\n", str[1]); // prints 'e'
printf("%c\n", str[2]); // prints 'l'
printf("%c\n", str[3]); // prints 'l'
printf("%c\n", str[4]); // prints 'o'
```
To print a string, we can use the `%s` format specifier:
```c
char str[] = "hello";
printf("%s\n", str); // prints "hello"
```
Note that the `%s` format specifier prints the entire string until the null character is reached.
We can also change individual characters in a string using array indexing:
```c
char str[] = "hello";
str[0] = 'H';
printf("%s\n", str); // prints "Hello"
```
In summary, a string in C is simply an array of characters terminated by a null character. We can initialize and access strings just like any other character array, and we can print them using the `%s` format specifier.